Authentication is a critical factor for all web applications, as it ensures that users are properly identified before being granted access. In the context of React application development, employing the right authentication process is essential for keeping data and users secure. Good authentication processes should strive to meet guidelines for reliability, flexibility, and scalability. From using two-factor authentication to enabling session locking after a pre-defined period of inactivity, these measures will help secure your application and keep user data safe.
In this article, we’ll explore 10 React authentication best practices that will help you secure your application and provide users with an enhanced experience.
Choosing the Right Authentication Method for Your React Application
The right authentication method can make or break a web application. With security in mind, it’s important to pick a methodology that is both effective and efficient. Options include token-based authentication, two-factor authentication (2FA), biometrics, OAuth, JSON Web Tokens (JWT), and more. While each has its pros and cons, any of them will ensure an extra layer of security for your users.
It is critical to assess the advantages and disadvantages of each one before making a selection. For instance, username-password combinations provide a basic level of security but are not always strong enough to protect sensitive information. On the other hand, multi-factor authentication requires more time and effort on the part of users but can be more effective in preventing unauthorized access.
It is also important to consider how each authentication method affects user experience when selecting one for your React application. Complex methods may slow down the login process or add too much complexity for some users, resulting in frustration or abandonment altogether. Furthermore, if an authentication system fails during login attempts due to technical issues, this could lead to further disruption in user experience.
React App Authentication: 10 Best Practices
React and the SPA pattern offer a unique approach to restricting user access – one that requires both backend and frontend implementations. By leveraging its powerful components, React can make it easier to control user permissions for specific parts of an application. This scenario is pretty commonplace for web applications, and utilizing React as part of the process enhances it significantly. Although challenging at times, implementing this approach with React guarantees solid security measures and truly tailored user experiences.
Securing data from unauthorized users requires dealing with potential threats both in terms of the application’s API and its front-end components. Talking about the front end, a user can access a view in one of two ways: by clicking on a link or by typing the URL into their browser. You can handle both cases properly if you:
- Prevent a link from being rendered. As part of an application’s security infrastructure, this practice ensures that only authorized and authenticated users can access restricted pages and data. To achieve this, server-side verification must be requested before any results are rendered on the client side. Only after the server has approved such verification is a link displayed. This way, unauthorized users can never view the specific page or content restricted by that link.
The use of straightforward conditional logic is the first method that springs to mind when you want to prevent a portion of your component from being rendered:
- Prevent a route from being accessible. This can be done by setting parameters within frontend routers that activate after successful verification with backend servers. Once those parameters are in place, routes or certain actions can only be accessed once a user is authenticated. This ensures unauthorized persons do not have access to confidential data or applications.
Using the example below, a user who tries to visit the /admin route without administrator authorization will immediately be sent to a prohibited page. All of that functionality is not required to be handled by the Admin /> component.
To ensure your React application is compliant with authentication standards, we’ve compiled the 10 best methods you should consider.
Utilize a Library
Ensuring your web app security is of paramount importance and using a library for authentication is one of the best practices. A library provides developers with useful solutions that have been vetted by other users, saving time and eliminating a lot of the guesswork in creating a secure authentication system.
Additionally, it’s easy to implement updates as they become available, which ensures that no loopholes are left unpatched and weak spots are secured. The library also allows you to tailor it specifically to your application’s needs, ensuring proper authorization control is in place. A good practice when selecting a library is to research its reviews and comments from those who’ve used it and find out how it works with your specific setup.
Avoid Storing Sensitive Information in Local Storage
One essential practice for React authentication is avoiding the storage of any sensitive information, such as passwords or personal information, in local storage. This way, companies expose the data to numerous threats, including malicious web pages that are specifically crafted to fetch and exploit stored data from the local system. A great alternative is storing sensitive user data on the server side or using tokens such as JWT.
The most effective way to protect user credentials is to store them on a secured server with limited access and encryption enabled. Using reliable back-end storage systems can also provide added assurances against potential theft and other cybercrime tactics.
Keep Your API Endpoints Secure
React developers must pay close attention when protecting their API endpoints, such as using HTTPS for requests and implementing client-side authorization controls in each endpoint. Additionally, the effective use of JWT tokens will add another layer of security by acting as proof that the server has authorized a request. An additional layer of security can be added with rate limiting and two-factor authentication solutions.
In addition, randomized session tokens should be implemented to prevent malicious parties from stealing user credentials and causing damage to sensitive information. Properly configured authentication processes can go a long way in ensuring that your platform remains secure and trustworthy.
Encrypt Passwords and Sensitive Data
The best practice for React authentication is to take advantage of powerful encryption algorithms that offer a strong level of protection and use multi-factor authentication whenever possible. This can be done through the use of AES or 3DES, which have been proven over time to protect important data from being accessed by those outside the designated user base.
Data can be encrypted using the built-in function “encrypt()” provided by React. You must provide both a key and the data you want to encrypt in order to use this function. The key is crucial since it is needed to both encrypt and decrypt the data.
Utilize HTTPS to Avoid the Man-in-the-Middle Attacks
Utilizing HTTPS for all web traffic helps encrypt data and prevent potential man-in-the-middle attacks from taking place. In these attacks, hackers attempt to intercept and modify communications between the user and server in an effort to access sensitive information like passwords. Allowing HTTPS communication can significantly reduce the chances of a successful security breach. Additionally, with HTTPS, you can guarantee the integrity of the data being passed between user and server, meaning that your users’ personal details will not be tampered with during login.
Limit the Number of Login Attempts
By tracking the number of login requests from a single IP address, it’s possible to determine if any suspicious activity is hinted at and, in turn, mitigate any potential threats to the user experience. Not only will this help keep users’ accounts secure, but it will also dissuade hackers looking to target your app or site. This is essential for protecting valuable and confidential data from unauthorized access.
You may, for instance, permit 5 login attempts every 5 minutes. An attacker must wait five minutes before attempting to brute force an account if they fail five times. This gives you enough time to recognize the attack and take appropriate action, including blocking the attacker’s IP address.
Use 2FA or MFA for Extra Security
Two-factor authentication (2FA) and multi-factor authentication (MFA) are increasingly being used to ensure added security in many popular applications. They provide an extra layer of defense against would-be attackers, allow organizations to set up more stringent rules for their accounts, and provide users with additional control over their own data. For example, when using 2FA or MFA to log into an application, oftentimes, passwords alone are not enough, and additional forms of authentication, such as biometric scanning, may be required.
By employing robust authentication techniques, businesses can protect their systems while also providing users with peace of mind by knowing that their private information is well protected from those who may seek to exploit it.
Avoid Using Email as a Username
To ensure maximum security of the user’s credentials, it is the best practice not to use their email addresses as usernames. This simple step can help protect user accounts from malicious actors trying to gain access. User names differ from emails in that they are generally shorter, more common, and easier for users to remember. Using a username system allows users to log into applications quickly and securely. Furthermore, combining a username with a strong password creates an extra layer of protection for the end user.
Email addresses are far more likely to be known to category-based hackers who target specific accounts with password-matching algorithms, making them more prone to being broken into. A unique username also helps protect users by obscuring their identity in databases, making it harder for criminals to do malicious activities such as linking other stolen login credentials with user accounts. Instead, creating a username specifically for the application you are developing can help deter attackers and better protect your users’ identity and privacy.
Employ Password-Free Authentication
Passwordless authentication has become the best practice for many React developers as it helps enhance security by removing the need to store passwords within user accounts. Passwords have become increasingly unreliable and challenging to manage securely. Therefore, passwordless authentication is a strong contender if you’re looking for an efficient and secure way of authenticating users. It’s easy to use, both for administrators and end users.
By leveraging mobile SMS code-based authentication, two-factor authentication, and other passwordless authentication options, developers can ensure that their applications offer the most secure experience possible to the end users. Moreover, there is no denying that passwordless authentication offers a much more hassle-free user experience than traditional username and password combinations.
Log off Inactive Users
In order to maintain the highest level of security for users’ information, the best practice for authentication is to log out inactive users regularly. This process is also known as “session timeout”. Session expiration provides extra layers of protection and ensures all users are regularly reminded to log off when leaving their computers unattended.
This can easily be done by setting timeout parameters that will force the user to re-authenticate if they have been inactive for an extended period of time. This helps ensure the security of user data, as accounts are logged out quickly and automatically before they become vulnerable to malicious actors.
Final Word
Access management presents one of the greatest challenges organizations face as they attempt to protect their physical infrastructure from potential threats. This challenge is no different when it comes to developing an application with React. Selecting an authentication method appropriate for your application can mitigate security risks and ensure that only authorized personnel have access to sensitive information.
Are you looking to move your business into the digital age? At Forbytes, we understand how difficult it can be to make the transition. That’s why we provide comprehensive software development services that cater to individual business needs. We pride ourselves on our ability to help companies all over the world develop new digital products, refine their business strategies, and introduce cutting-edge innovation into their domain. Reach out to us today; our team of professionals will be more than happy to assist you in creating a successful app tailored to your business needs.
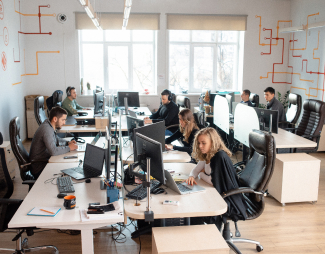
Our Engineers
Can Help
Are you ready to discover all benefits of running a business in the digital era?
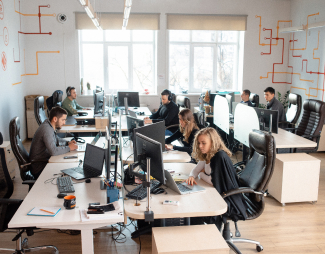
Our Engineers
Can Help
Are you ready to discover all benefits of running a business in the digital era?